Project: Mobilize
Mobilize is a organizing and planning desktop application used for teaching Software Engineering principles. The user interacts with it using a CLI, and it has a GUI created with JavaFX. It is written in Java, and has about 6 kLoC.
Code contributed: [Functional code] [Test code]
Enhancement Added: Detag
Removing tags from multiple contacts : detag
When a tag is no longer in use, you can use the detag command to remove the tag from multiple contacts.
Format: detag INDEX… [t/TAG]
Examples:
-
list
detag 2 t/friends
Deletes the [friends
] tag of the 2nd contact in the address book. -
find Betsy
detag 1, 2, 4 t/OwesMoney
Deletes the [OwesMoney
] tag of the 1st, 2nd and 4th contact in the result list of the find
command.
Constraints
Note the following constraints when trying to remove a tag:
-
INDEX refers to the index number shown in the most recent listing.
-
The index must be a positive integer 1, 2, 3, …
-
Every index must be separated by a comma.
End of Extract
Justification
With many contacts added to the application, when one specific tag is no longer in used, it will be troublesome for the users to remove tag from the contacts individually.
Hence, by specifying the tag to be removed and the contacts having the tag, users can determine multiple contacts to be removed with the tag. The command requires specifying of contacts so that it can function in the situation where the tag is currently still using but only certain group of contacts does not need it.
Detag mechanism
The detag mechanism is facilitated by the DetagCommand
class which is inherited from the UndoableCommand
class. It allows for the removal of existing tags from multiple contacts, i.e. detag. The minimum number of contacts that can be specified is 1 and the maximum number is the number of contacts in the current list.
Suppose the user executes the command detag
using the following code:
`detag 1, 2 t/friends`
The DetagCommandParser
class will check for the validity of the indices specified, i.e. within the range of the filtered list. Afterwards, the LogicManager
invokes the method deleteTag
, which removes the specific tag from the Set
of tags from the specified contacts, and updates the storage.
If one or more of the contacts specified by the indices does not contain the specified tag to remove, the removal will not be executed even for those contacts that have the tag. |
The current detag mechanism does not support multiple tag removal, i.e. remove 2 different tags in one command line. |
Design Considerations
Aspect: Implementation of DetagCommand
Alternative 1 (current choice): Directly removes the tag from Set
of contact(s)
Pros: Easier and more specific command to remove a tag, and allows removal from multiple contacts in one command
Cons: Introduction of new command specifically for the removal of a tag may not be necessary.
Alternative 2: Just use the EditCommand
to change the tag
Pros: Use of one command to edit any details of a person is more organised.
Cons: Cannot not remove tags of multiple contacts, and it is mandatory to retype the tags that do not need to be removed.
End of Extract
Using the calendar
A calendar is useful for marking important dates. When you have many deadlines and birthdays to take note of, the calendar allows you to view all these important dates at a glance.
Marking dates
With many contacts and tasks to manage, differentiating between birthdays and deadlines on the calendar can be done with the help of colours.
Dates are marked automatically when a contact/task is added, edited or deleted. |
Examples:
-
A task has a deadline on
17 July 2017
.
The corresponding date on the calendar is marked red. -
A contact has his/her birthday on
09 December 2017
.
The corresponding date on the calendar is marked pink. -
17th July 2017
is both a task’s deadline and a contact’s birthday.
The corresponding date on the calendar is marked yellow.
Constraints
Note the following constraint on the colour of a date:
Viewing deadlines and birthdays
When there are many deadlines or birthdays on the same date, instead of switching between CommandMode
and using the find
command, you can just click on the date itself.
Examples:
-
17
on the calendar that represents17th July 2017
is being clicked.
Returns a list of tasks with deadlines on 17th July 2017 and a list of contacts with birthdays on 17th July 2017, respectively.
Constraint
Note the following constraints when clicking a date on a calendar:
End of Extract
Justification
With many tasks and contacts added to the application, it is useful to have a calendar interface for the user to interact and view the overview of upcoming important events.
Hence, with the marking of dates with colour on the calendar, users can easily identify what is upcoming and be more prepared.
The returning of tasks list and contacts list afflicted with the date clicked is also important as users can easily find out what is happening on that specific date with just one click. Thus, saving users' time and effort to conduct find
on contacts list and tasks list.
Calendar mechanism
The calendar mechanism is facilitated by the DatePickerSkin
skin instance which capture the pop-up content from the DatePicker
control in JavaFX
. It allows the user to have a calendar view of all contacts' Birthday
and tasks' Deadline
.
Suppose the address book is modified (e.g. using add
, edit
, addtask
, deletetask
).
After the modification of Mobilize’s address book (i.e. saving of data to the addressbook.xml
file), ObservableList
of Person
and Task
classes stored in the address book are passed into the CalendarPanel
Ui through Model
.
Next, getDayCellFactory
will format the DateCell
of the DatePicker
. The formatting is done with birthday and deadline as conditions and colour and tooltip of DateCell
as variables to be change by the formatter.
By selecting the date on the calendar, the date value from the DatePicker
will be captured and be parsed as a string. The string is then passed to Logic
to execute the FindCommand
which will show all contacts and tasks having Birthday
and Deadline
on that specific date.
[NOTE] Implementation of CLI for the calendar is still ongoing.
The following are examples of colour and tooltip of the calendar:
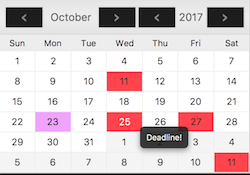
Figure 4.11.a: Tooltip and colour for Deadline
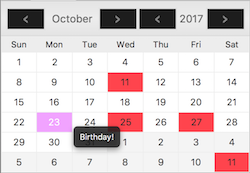
Figure 4.11.b: Tooltip and colour for Birthday
Design Considerations
Aspect: Implementation of Calendar
Alternative 1 (current choice): Use DatePickerSkin
to get the calendar look and function.
Pros: Less coding needed as the template, DatePicker
, is already available. Thus, more time can be spent on other functions related to the calendar.
Cons: Overall look is fixed.
Alternative 2: Design a calendar using JavaFX
Pros: Fully customizable.
Cons: Much effort required to ensure it is bug free.
Aspect: Loading of data to Calendar
Alternative 1 (current choice): Use address book data that is passed to the CalendarPanel
through Model
Pros: Able to retrieve updated data from UniquePersonList
and UniqueTaskList
that are already available.
Cons: Increase coupling with Person
and Task
.
Alternative 2: Create a separate UniqueMarkDateList
that stores required dates with relevant information (e.g. Person’s name and Task’s description)
Pros: Reduces coupling as the data will be stored during initialisation of address book and addition/deletion of person/task.
Cons: We must ensure that the data stored is bound to the individual person and task, such that editing of such person/task will be reflected.
End of Extract
Enhancement Proposed: Commandline interface for calendar and adding of deadlines/birthdays directly through the calendar
Justification
As Mobilize is designed to be a Commandline interface application, interaction with the calendar should also be done with just the commandline.
Possible idea
One possible idea is the addition of Calendar
mode to the current AddressBook
and TaskManager
mode. Under this mode, possible commands should include controlling the display month and year, controlling the current selected date with the arrow keys and adding of deadlines or birthday directly through the calendar.
This idea allows for more convenience offered to the users when interacting with the application and will definitely save time and effort to organise and look through the upcoming events.